The software development world increasingly relies on .NET developers, making understanding its essential concepts critical for beginners. Hiring teams often evaluate candidates through challenging .NET interview questions, focusing on concepts like the .NET Framework, web services, and application development. Excelling in these interviews ensures a competitive edge in software development. Whether the role involves building desktop applications, creating web applications, or managing database connections, mastering these questions is essential.
Aloa is a software agency that leverages a robust project management framework to deliver high-quality net applications. Our network of vetted partners ensures clients work with top .NET developers using the latest industry tools, such as Visual Studio and advanced programming languages. We prioritize seamless project execution, from code reuse to managing data sources and crafting optimized user interfaces. A dedicated product owner oversees your project, focusing on your business needs and goals.
Leveraging our extensive experience, we created this guide to explore essential .NET interview questions. By the end, you’ll confidently navigate building applications for different operating systems and compatible languages, ensuring your readiness for real-world projects.
Let's get started!
Basics of .NET Interview Questions on the .NET Framework
Understanding the .NET Framework is crucial for tackling common .NET interview questions. These questions often focus on foundational concepts like the Common Language Runtime, base class libraries, and the difference between managed and unmanaged codes. Here are three .NET framework basics to ask and master.
1. What is .NET, and what are its key components?
.NET is a versatile development platform designed for building and running different applications, including web, desktop, and mobile. It supports multiple net languages like C#, Visual Basic, and F#, enabling developers to create high-performance solutions across platforms. The platform compiles source code into an intermediate language (IL), which the virtual machine processes during runtime to execute native machine code.
Key components of the .NET Framework include:
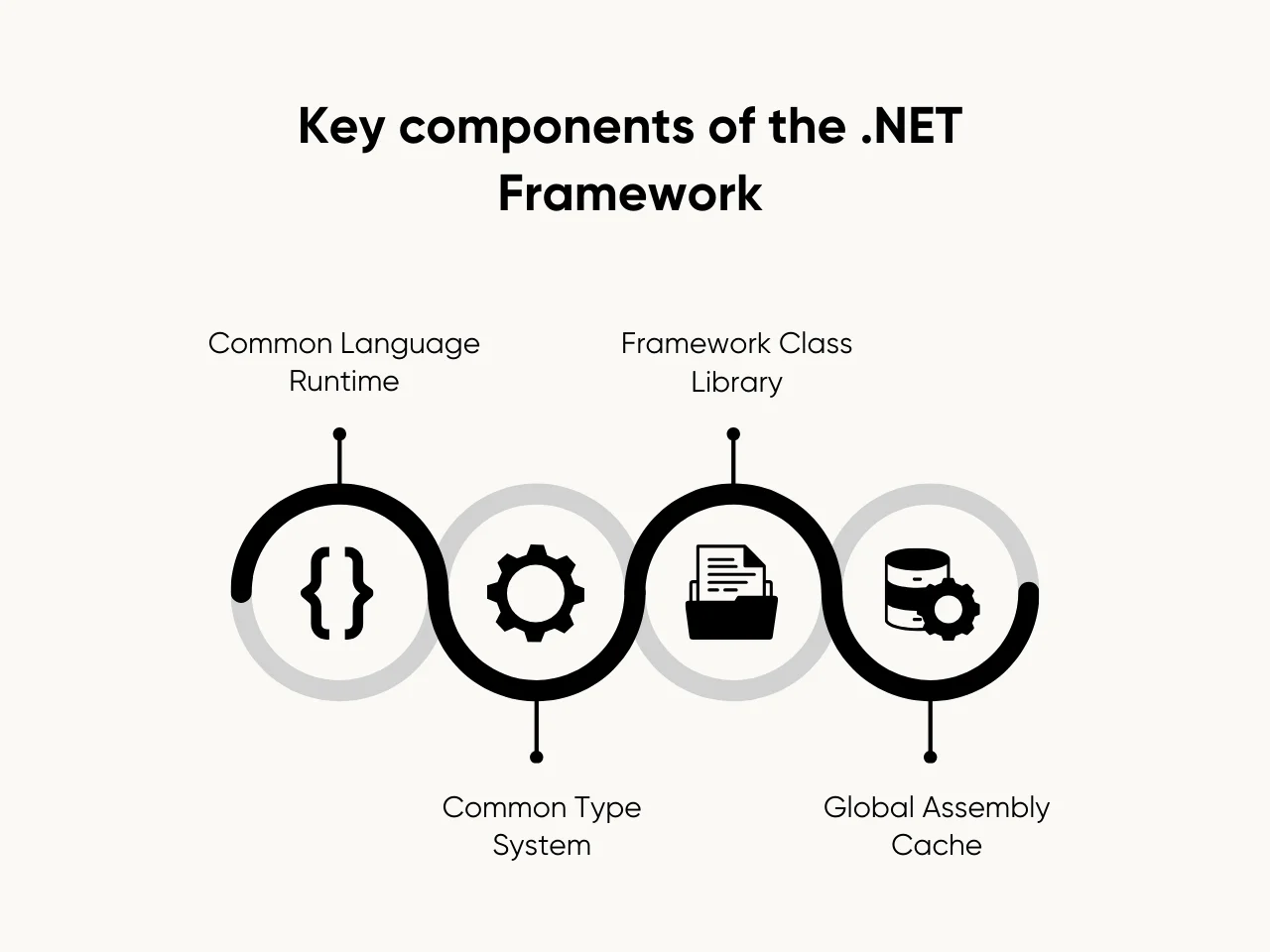
- Common Language Runtime (CLR): This runs garbage collection, memory management, and thread management. It also ensures secure execution through features like code access security and exception handling.
- Common Type System (CTS): This system provides a set of rules to ensure type compatibility across net languages during the development process.
- Framework Class Library (FCL): A rich library for user input, business logic, and interactions like command line operations.
- Global Assembly Cache (GAC): Manages types of assemblies and stores shared libraries for different applications.
The .NET Framework simplifies the development process. Its components, like garbage collector, common language specification, and intermediate code, ensure efficient run time and secure execution. This platform transforms code into optimized native language, allowing seamless interaction between user interaction and backend systems, whether working on web browsers or other platforms.
2. Explain the difference between .NET Framework, .NET Core, and .NET 5/6
The .NET Framework, .NET Core, and .NET 5/6 are critical platforms for developers working on .NET interview questions. These technologies ensure the smooth execution of managed code through the execution engine. Understanding their differences is essential for navigating interview discussions.
.NET Framework, .NET Core, and .NET 5/6 differ in architecture, functionality, and scope. Below is a detailed comparison:
These platforms illustrate the different types of development possibilities. Developers have many options, from creating multipurpose Internet mail extensions (MIME) applications with mime header management to supporting advanced compiled code.
Mastering these differences allows developers to adapt to evolving technologies. Leveraging .NET Core for cloud-ready apps or adopting .NET 5/6 for cutting-edge solutions showcases adaptability and competence in .NET interview questions.
3. What are Common Language Runtime (CLR) and Common Type System (CTS)?
The Common Language Runtime (CLR) and Common Type System (CTS) form the foundation of the .NET Framework. Understanding these concepts is essential to answering .NET interview questions effectively. They ensure smooth execution and compatibility of applications developed using .NET languages.
These are the core components of the .NET Framework:
- CLR: It executes code and manages system services. It handles critical tasks like memory allocation, garbage collection, and exception handling, ensuring efficient application performance.
- CTS: It maintains type compatibility across programming languages. Developers can define types in one language and use them seamlessly in another, making .NET a versatile and interoperable platform.
The CLR and CTS enable developers to create robust, language-independent applications. Mastering their features is vital when preparing for dotnet interview questions. Highlighting these concepts during interviews showcases your understanding of the .NET Framework's architecture and capabilities. Focusing on such fundamentals ensures you can confidently tackle questions and impress hiring managers with your expertise.
Object-Oriented Programming (OOP) in .NET
Object-Oriented Programming (OOP) is central to understanding .NET interview questions. Employers often evaluate your grasp of principles like encapsulation, inheritance, and abstraction. Mastering concepts such as abstract classes, delegate objects, and data manipulation ensures you can design robust, scalable solutions. Let’s dive into these essential OOP topics.
4. How does .NET support Object-Oriented Programming?
.NET fully supports object-oriented programming (OOP), making it easier for developers to create modular and reusable code. OOP principles form the foundation for designing robust and maintainable software solutions. In .NET, developers can apply these principles effectively using languages like C# and VB.NET.
These are the core principles of OOP in .NET:
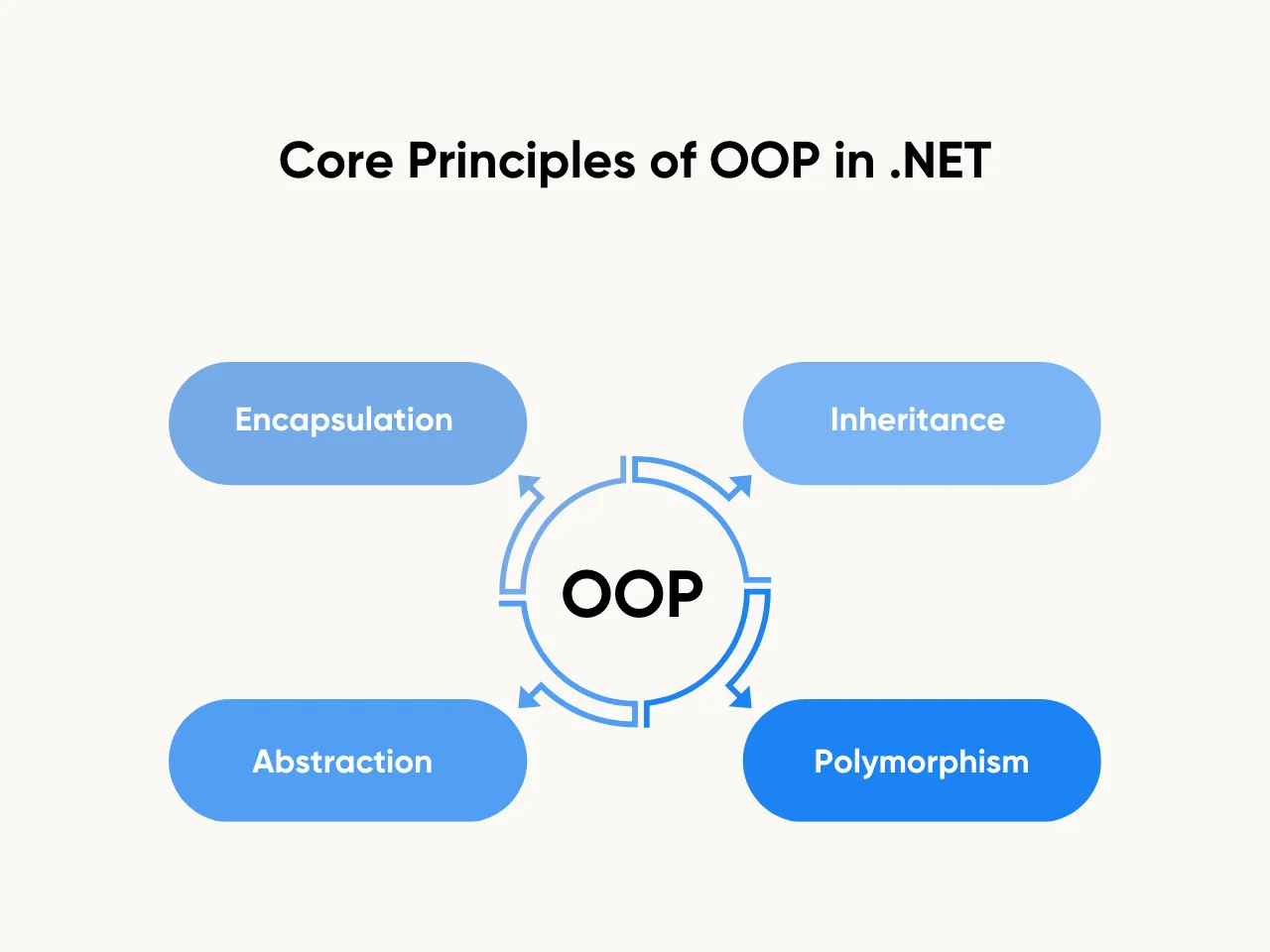
- Encapsulation: Ensures an object’s internal state is hidden from external modification. Developers use access modifiers such as private and protected to restrict access to data, allowing controlled interaction through methods.
- Inheritance: Enables the creation of new classes from existing ones. A base class provides common functionality, while derived classes add or override specific behaviors, promoting code reuse.
- Polymorphism: Allows methods to perform differently based on the object’s type. Using method overriding or interfaces, developers can design flexible and extensible code that adapts to varying requirements.
- Abstraction: This approach focuses on exposing only the essential features of an object. Developers define abstract classes or interfaces, ensuring objects adhere to a specific contract while hiding unnecessary implementation details.
.NET’s strong alignment with OOP principles empowers developers to write efficient and scalable code. Encapsulation protects data integrity, inheritance promotes reuse, polymorphism enhances flexibility, and abstraction simplifies complexity. Mastering these principles ensures you are well-prepared for any .NET interview questions, showcasing your understanding of object-oriented programming.
5. What is the difference between an abstract class and an interface in .NET?
Abstract classes and interfaces have distinct characteristics. An abstract class can include both implemented methods and fields. Developers use them to define shared functionality that subclasses can inherit and extend. On the other hand, an interface strictly defines method signatures and properties without implementation. It allows multiple classes to implement shared behaviors while maintaining loose coupling.
Choosing between an abstract class and an interface depends on the application's design. Use an abstract class when shared code or default behaviors are necessary across derived classes. Opt for an interface when you want to define a contract that multiple unrelated classes can implement. Understanding these differences helps developers structure their code effectively and ensures a strong grasp of core concepts, often a focus in .NET developer interview questions and asp.net interview questions.
6. What is method overloading and overriding in .NET?
Method overloading and overriding are essential concepts in .NET interview questions. Method overloading allows multiple methods with the same name but different parameters to coexist. It provides flexibility in designing methods to handle varied inputs. Method overriding, on the other hand, enables a derived class to modify the behavior of a base class method. This is achieved using the override keyword and ensures runtime polymorphism in OOP.
Here are the differences between method overloading and overriding in .NET:

Method overloading and overriding have practical applications in .NET projects. Overloading is often used to create flexible methods like constructors that accept varying parameters. Overriding is critical for implementing polymorphism, ensuring specific behavior in child classes while retaining the parent class’s structure. Mastering these concepts is crucial for solving .NET interview questions effectively.
C# Programming Basics
A solid understanding of C# programming basics is essential to answering .NET interview questions effectively. Topics like value types, reference types, and data structures highlight your programming foundation. Knowing how to use data types, handle runtime execution, and manage source code can set you apart. Here’s what to focus on.
7. What are value types and reference types in C#?
Value and reference types are fundamental concepts in C# and frequently appear in .NET interview questions. Value types directly store data in memory, while reference types store a reference pointing to the data's memory location. These types are crucial in understanding memory management and data handling in C# programming.
Here are the differences between value types and reference types in C#:
The distinction between value and reference types has practical implications for memory management and performance in C#. Value types offer better performance because their stack-based allocation reduces overhead. However, they have a limited scope and disappear once the method is completed. Reference types provide flexibility, especially for managing complex data structures, but they increase the risk of memory leaks if incorrectly handled.
8. What is the var, dynamic, and object role in C#?
C# provides flexible type declarations using var, dynamic, and object, catering to different development needs. These keywords play essential roles in simplifying type management while maintaining functionality and adaptability in code.
These are the key roles of var, dynamic, and object:
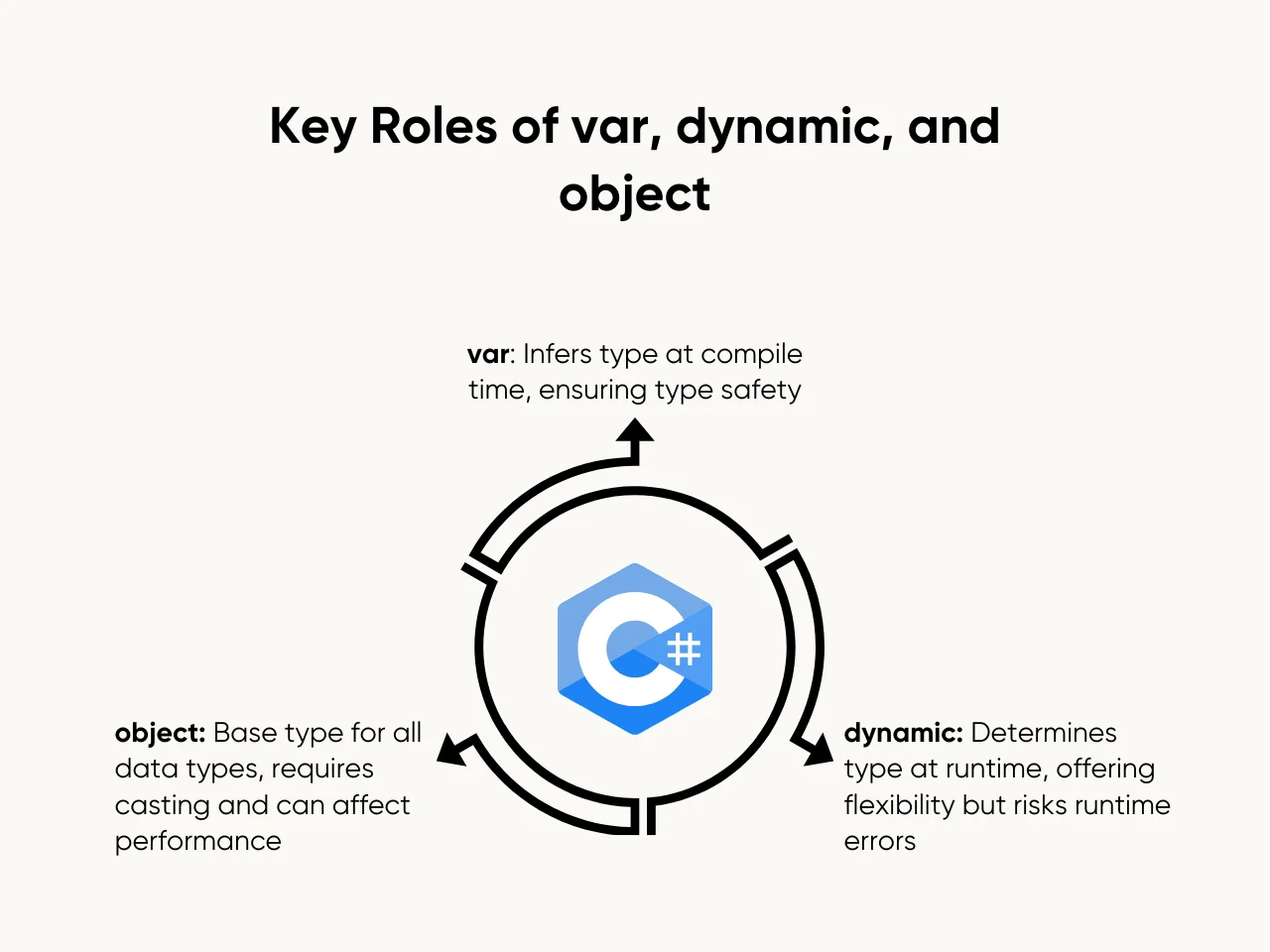
- var: Infers the type during compile time based on the value assigned. It ensures type safety while allowing concise syntax. For instance, a var variable cannot change its type once assigned, promoting consistency.
- dynamic: Determines the type at runtime, offering greater flexibility when the type is unknown during compilation. However, this flexibility comes with the risk of runtime errors if the type is incorrectly handled.
- object: Acts as the base type for all data types in C#. It is highly versatile, allowing storage of any value, but requires explicit type casting when retrieving data, which can impact performance.
var, dynamic, and object provide unique capabilities for handling types in C#. While var ensures simplicity and clarity during compile time, dynamic offers runtime adaptability, and object serves as a universal type placeholder. Familiarity with these roles helps developers write efficient and versatile code, a key skill for confidently tackling .NET developer interview questions.
9. What are nullable types in C#?
Nullable types in C# allow value types, which typically cannot hold null values, to represent null. This feature is helpful for scenarios where a variable might not always have a value, such as database operations or optional parameters. Adding a ? to the value type declaration creates a nullable type. For example, int? age = null; the variable age can store an integer or a null value.
Nullable types provide flexibility in handling data and help prevent runtime errors. Developers can use nullable types to signify the absence of a value explicitly, making the code more expressive and robust. This capability is essential in applications dealing with dynamic data or user inputs, where values might need to be added or defined. Understanding nullable types is crucial for answering .NET interview questions, as they highlight your ability to write adaptable and error-resistant code.
Application Development with .NET
Application development lies at the heart of .NET interview questions. Proficiency in creating web applications, handling data access, and managing database connections is vital. Key skills include leveraging the Entity Framework, implementing web pages, and building user-friendly user interfaces. Here’s what every .NET developer needs to know.
10. What is ASP.NET, and what are its key features?
ASP.NET serves as a powerful framework for building dynamic web applications. Developers use it to create high-performance websites and services tailored to various business needs. The framework offers flexibility, scalability, and a wide range of tools, making it a popular choice in application development.
These are the key features of ASP.NET:

- MVC Pattern: ASP.NET supports the Model-View-Controller (MVC) architecture, which separates application logic, user interface, and data management. This separation improves code maintainability and testability.
- Razor Syntax: The Razor view engine simplifies embedding server-side code within HTML. This approach enhances code readability and reduces development time.
- Support for RESTful APIs: ASP.NET allows seamless development of RESTful APIs, enabling smooth communication between client and server. Developers can create scalable, secure, and efficient APIs for various platforms.
ASP.NET provides developers with the tools and frameworks necessary for modern web development. The MVC pattern encourages clean architecture, Razor syntax simplifies coding, and RESTful API support ensures smooth integration. Mastering these features equips you to handle advanced .NET interview questions, demonstrating your capability in application development.
11. What are Web APIs, and how are they implemented in .NET?
Web APIs in .NET are frameworks designed to build HTTP-based services that various clients can consume, including web browsers, mobile apps, and desktop applications. They facilitate communication between applications through standard HTTP methods like GET, POST, PUT, and DELETE, making them essential for creating RESTful services. ASP.NET Web API, a key component of the .NET ecosystem, simplifies the process of building these APIs while ensuring scalability and performance.
To implement Web APIs in .NET, developers typically define controllers to handle requests and responses. For example, a basic GET endpoint retrieves data from the server, while a POST endpoint allows data submission. Using ASP.NET Web API, developers can quickly set up these endpoints with clear routing and middleware configurations.
12. What is Entity Framework (EF) in .NET?
Entity Framework (EF) is an Object-Relational Mapping (ORM) tool that simplifies database interactions in .NET applications. It allows developers to work with databases using .NET objects, eliminating the need for extensive SQL code. EF enhances productivity and streamlines database operations.
Here are the key features of Entity Framework include:
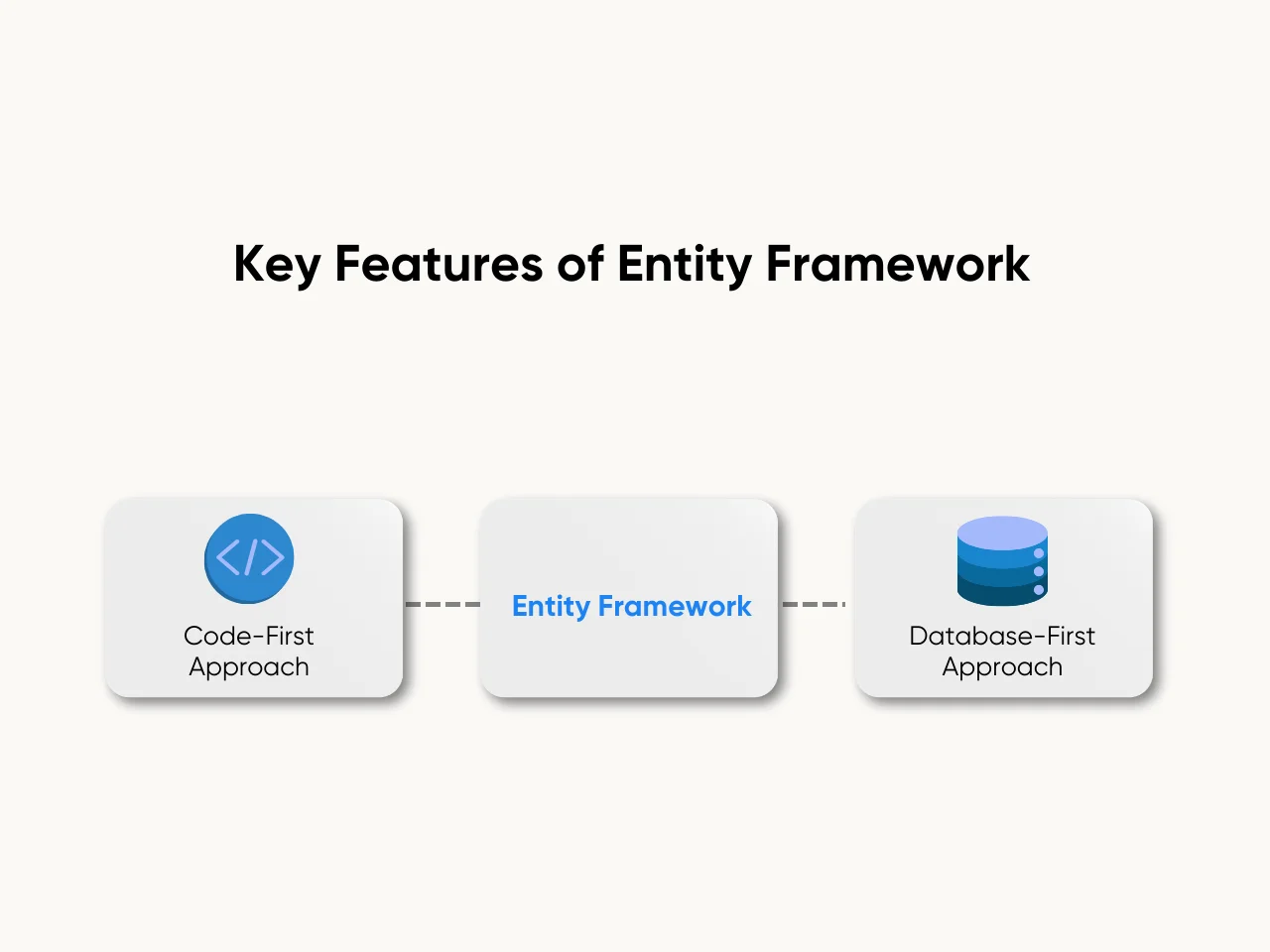
- Code-First Approach: Developers define the database schema using C# or VB.NET classes. EF then generates the database based on these definitions, offering flexibility in managing schema changes through migrations.
- Database-First Approach: Ideal for existing databases, EF generates .NET classes based on the current database schema. This approach helps developers integrate legacy databases seamlessly into their applications.
Consider creating a simple model using the Code-First approach. Define a class named Product with properties like ID, Name, and Price. With EF, you establish a connection to the database and use a DbContext class to query and save data. The framework handles the translation of LINQ queries into SQL commands, simplifying data access.
Entity Framework is a powerful tool for efficiently managing data operations in .NET. Whether building new databases with the Code-First approach or integrating existing ones using Database-First, EF significantly reduces development time. Mastering EF prepares developers to tackle advanced .NET interview questions related to data management.
Key Takeaway
Preparing for .NET interview questions helps candidates showcase their expertise in essential concepts. During interviews, companies often assess knowledge of the .NET Framework, object-oriented programming, and application development. Understanding these topics ensures beginners can confidently demonstrate their skills and secure their desired roles.
When hiring, companies should evaluate applicants' understanding of .NET interview questions, focusing on technical skills and application development experience. By exploring the best sites to hire mobile app developers, businesses can streamline their search for top talent. A structured approach ensures you find developers ready to meet your project's demands.
Are you looking to ace your next interview or find the perfect .NET developer for your team? At Aloa, we guide you through every step of software development, connecting you with highly skilled teams ready to build your vision. Start your journey to success with Aloa’s expert network and tools.